你可以通过部件功能模块扩展嬴图Manger的功能。开发人员可以在此实现自定义应用和解决方案的开发。熟悉UQL的用户还可以通过用于执行特定语句的部件而受益。
用户可以在嬴图Manager中方便地访问发布的部件,或将其嵌入到任何其他网页中使用。
代码编辑器
部件的代码编辑器包括三部分:Template(HTML),Style(CSS)和Script(JavaScript)。
当前版本的部件不支持使用多个HTML、CSS或JavaScript文件。
Template
Template部分使用Handlebars模板,这部分代码会合并到最终HTML输出的<body>
标签中。当前版本不提供对其他标签的访问。因此,在这部分代码中无需包含DOCTYPE
、<html>
、<head>
或<body>
等元素。
Handlebars使用双花括号{{ }}
来表示在渲染过程中将被替换为实际值的表达式。
<h1>{{text}}</h1>
<!-- Value of the variable 'text' is set in the script -->
Style
Style部分使用Less作为CSS预处理器。这部分代码会包含在最终HTML输出末尾的<head>
标签中。
body {
font-family: system-ui;
background: #eff3f4;
color: #00B0F0;
text-align: center;
}
Script
在Script部分中,初始步骤应该为:
- 导入模板和样式
- 使用适当的脚本逻辑定义并实例化
ModuleInterface
的子类
1. 使用自定义布局渲染
import "./index.less"
import template from "./index.hbs"
const { ModuleInterface } = require('@/core/module.interface')
class ManagerModule extends ModuleInterface {
constructor(name, template) {
super(name, template)
}
init() {
console.log("Initialized init",this.__name)
}
async execute() {
// Set the value of the variable 'text' contained in the template
this._render({ text: "My first widget!" })
}
}
window._managerModule = new ManagerModule("helloUltipa", template)
结果预览:
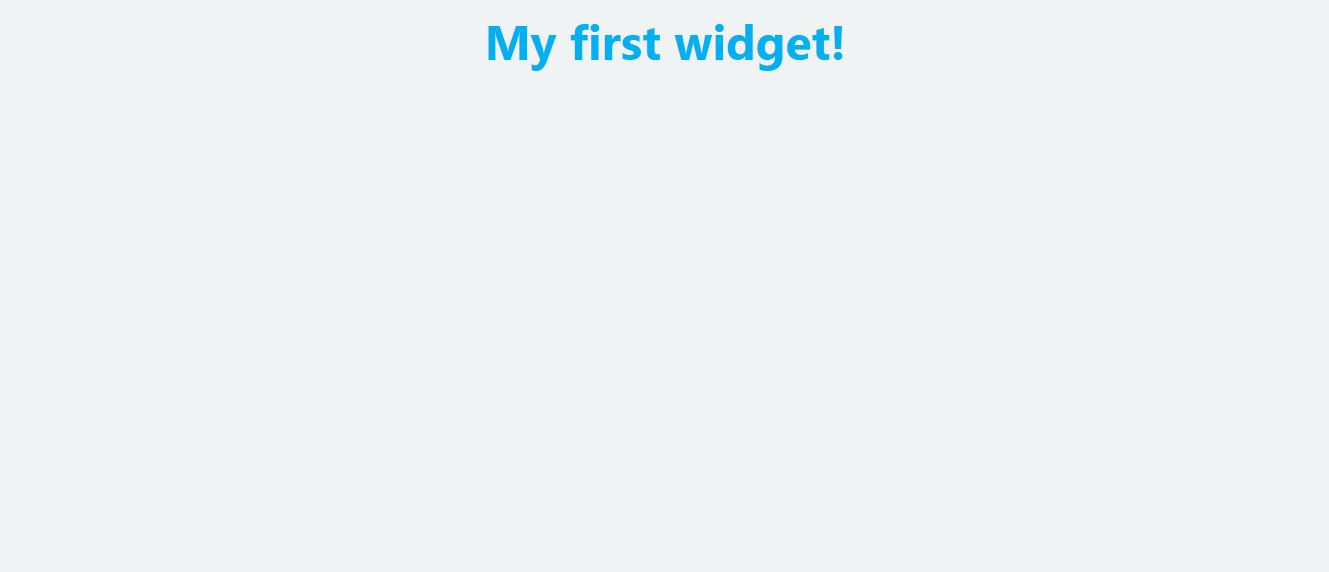
2. 使用默认布局渲染
大多数情况下,当想要发送UQL并接收服务器响应时,必须利用Client
类的实例并使用默认的嬴图布局呈现查询结果。
import "./index.less"
import template from "./index.hbs"
const { ModuleInterface } = require('@/core/module.interface')
const { Client } = require('@/core/utils')
class ManagerModule extends ModuleInterface {
constructor(name, template) {
super(name, template)
}
init() {
console.log("Initialized init",this.__name)
this.client = new Client()
}
async execute() {
const resp = await this.client.uql('n().e().n() as p return p{*} limit 10')
this._renderUltipaData(resp)
}
}
window._managerModule = new ManagerModule("helloUltipa", template)
结果预览:
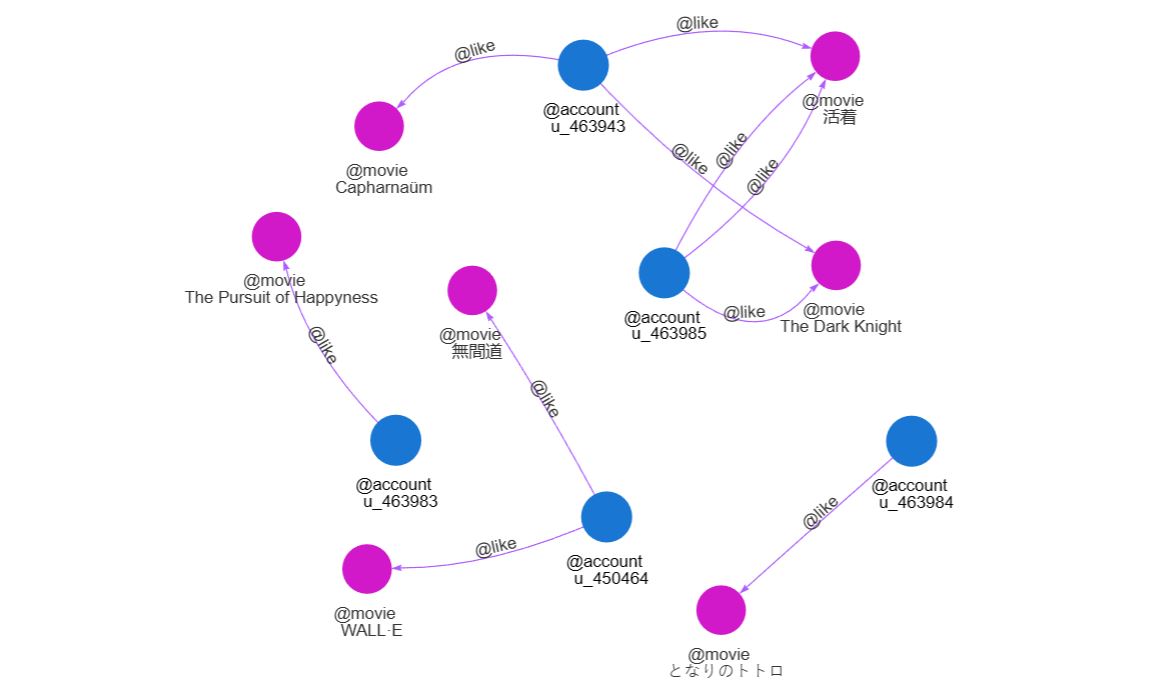
请留意:此例从当前图集获取10条路径,并在2D布局上应用当前设置的样式。因此,你运行的结果可能不一样。
如果你打算使用默认布局渲染不是从嬴图数据库中检索到的数据,请确保你的数据结构符合嬴图服务器的响应格式,然后再将其传递到
_renderUltipaData()
。
3. 导入第三方库
以下是Script编辑器默认加载的依赖项:
dependencies:{
"3d-force-graph": "^1.73.0",
"@cosmograph/cosmos": "^1.4.1",
"@googlemaps/polyline-codec": "^1.0.28",
"@turf/turf": "^6.5.0",
"@ultipa/fetch2": "^0.0.7",
"ag-grid-community": "^30.2.1",
"axios": "^1.6.1",
"echarts": "^5.4.3",
"handlebars": "^4.7.8",
"highcharts": "^11.2.0",
"highlight.js": "^11.9.0",
"json-formatter-js": "^2.3.4",
"jstoxml": "^3.2.10",
"leaflet": "^1.9.4",
"lodash": "^4.17.21",
"moment": "^2.29.4",
"vis-data": "^7.1.9",
"vis-network": "^9.1.9",
"vkbeautify": "^0.99.3"
}
使用其中的一些项目:
import * as echarts from 'echarts'
import axios from 'axios'
如果要使用其他的库或框架,请在初始化期间异步加载外部JavaScript文件:
...
class ManagerModule extends ModuleInterface {
...
async loadJS(url) {
let response = await axios(url)
eval(response.data)
}
async init() {
await this.loadJS("https://code.jquery.com/jquery-3.6.0.min.js")
this._render()
...
}
...
}
参数表配置
构建参数表
你可以将需要用户输入的变量集成到表单中,并将变量值传递给脚本。
对于每个变量(字段),需要配置以下内容:
项目 |
描述 |
要求 |
---|---|---|
Variable | 变量名称 | 必须与脚本中使用的变量名称一致 |
Label | 变量标签 | / |
Placeholder | 输入变量的占位文字 | / |
Type | 变量类型 | 有些类型提供进一步的配置 |
变量类型包括:
类型 | 数据类型 |
---|---|
String | string |
Number | number |
Node_Input | string (node ID) |
Radio | string |
CheckBox | []string |
Select | string or []string |
Schema | string |
Property | string |
Date Time | string |
UQL | string |
在脚本中使用变量
你可以将formData
传递到execute()
函数中,以formData?.<variable_name>
的格式指定具体的变量:
...
class ManagerModule extends ModuleInterface {
...
async execute(formData) {
// The variable name in this example is 'limitNo' with the type Number; set the default as 1
const resp = await this.client.uql(`find().nodes() as n return n{*} limit ${
formData?.limitNo || 1
}`)
this._renderUltipaData(resp)
}
...
}
编译,测试,发布
编译
在测试或发布部件之前,需要进行编译。有任何代码修改都需要再次编译。
测试
点击测试,运行部件。如需输入参数,请点击测试按钮旁的齿轮图标。
在结果预览窗格查看部件测试运行的结果。如果脚本中包含任何UQL,请留意它是在当前图集上运行的。
另外,使用默认布局渲染时,结果预览窗格仅使用默认样式。如需应用其他自定义样式,请在发布部件后,点击UQL编辑器旁的部件图标,找到该部件后运行。
发布
点击UQL编辑器旁的部件图标,可以查看所有已发布的部件。修改部件后,请重新发布。
设置
点击部件名称旁的配置图标,就可以编辑部件的名称、描述和缩略图(建议尺寸180px*180px)。打开<iframe>选项后,可以将相应的代码嵌入到其他网页中后,到期前都可以在该网页中使用这个部件。<iframe>
元素的src
属性包含以下参数:
参数 |
描述 | 配置 |
---|---|---|
shortCutId | 部件ID | / |
params | 所有参数以及在测试表单中设置的参数值 | / |
theme | 显示主题 | light , dark |
colorStyleSelected | 当前选择的结果展示样式 | / |
shareToken | 为了能够在其他网页使用部件而生成的Cookie | / |
当关闭<iframe>选项又重新打开,或修改到期日期时,会重新生成<iframe>
元素。
导入/导出部件
用户可以将部件导出为ZIP文件,并在需要时重新导入至嬴图Manager。